Recently, I am working on a project that requests to use HTTPS in order to communicate with Amazon S3 (cloud storage). I did the research on how to install SSL on my localhost and here is my solution.
Important note:
This tutorial is to create a self-signed certificate. At the end of this post, you should be able to use HTTPS on your localhost. When you use HTTPS on your browsers, you will see a “not secure” warning as the screenshot below. What you do, you just hits on “Advanced button” (1) and hits on “Continue to yourdomain (unsafe)” link (2). The HTTPS will continue to be red as the screenshot below on your localhost. Because it is a self-signed certificate.
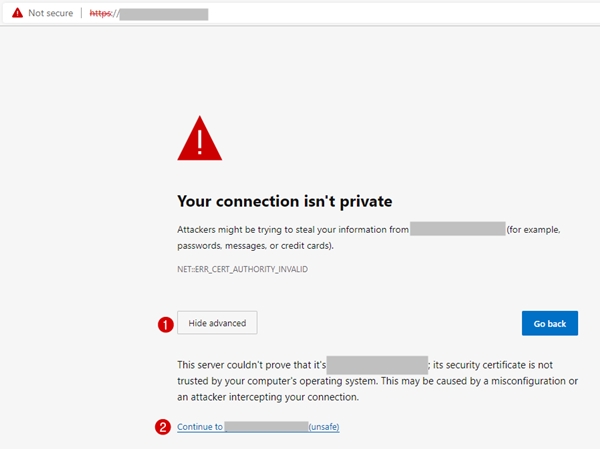
Install WampServer
In this tutorial, we will install SSL on WampServer. It should work on other PHP servers as well such as Xampp, MAMP, and AppServ. I personally use WampServer.
Install OpenSSL
Next, we will install OpenSSL. Download and install OpenSSL on your computer. I use Win64 so I download the Win64 OpenSSL full version. I install the OpenSSL in “E:\OpenSSL-Win64“. You install it anywhere on your computer.
Create your key and certificate
On the localhost, we will create our self-signed SSL certificate. To do that, open your command line(CMD) as Administrator. You can use any terminal you like but it needs to open with the Administrator user.
At the command prompt, change the current directory to your OpenSSL directory where you install it. In my case, I install OpenSSL in “E:\OpenSSL-Win64“. So I will change the directory as code below.
cd E:\OpenSSL-Win64\bin
Private key
Create our private key by the command line. This step will ask you for a passphrase. You can make up any passphrase you want.
Now, run the command line below to generate your private key.
openssl genrsa -aes256 -out private.key 2048
Then run another command line.
openssl rsa -in private.key -out private.key
Certificate
Create our certificate. This step will ask you some questions. You can leave the default answers (just hit Enter without typing anything) except the Common Name question. The answer to the Command Name question must be localhost. So you must type the localhost for the Command Name question.
Now, run the command line below.
openssl req -new -x509 -nodes -sha1 -key private.key -out certificate.crt -days 36500
After running this command line, you already generated the certificate.crt and private.key files. These files are stored in the OpenSSL folder where you install OpenSSL. In my case, it is “E:/OpenSSL-Win64“.
Move your private key and certificate files
We will move the private key and certificate files that we just create into the Apache folder.
Create a new “key” folder in Apache folder
To do that, we will create a new folder called “key” under your Apache folder (E:\wamp64\bin\apache\apache2.4.46\conf).
Mine looks like this E:\wamp64\bin\apache\apache2.4.46\conf\key
Move your private key and certificate files to the key folder
Go to “E:\OpenSSL-Win64\bin” where you run the command line to generate the private.key and certificate file.
In “E:\OpenSSL-Win64\bin“, you will find the private.key and certificate.crt that you created. Move these two files into the key folder ( E:\wamp64\bin\apache\apache2.4.46\conf\key ).
Now in the key folder, you will have both private.key and certificate.crt.
Edit your httpd.conf
In your httpd.conf (E:\wamp64\bin\apache\apache2.4.46\conf), find and uncomment the lines below. uncomment means to remove “#” in front of the line.
LoadModule ssl_module modules/mod_ssl.so
Include conf/extra/httpd-ssl.conf
LoadModule socache_shmcb_module modules/mod_socache_shmcb.so
We do it to tell Apache to load the SSL module and load the httpd-ssl.conf which we will edit in the next step.
Edit your httpd-ssl.conf
This step will edit the httpd-ssl.conf (E:\wamp64\bin\apache\apache2.4.46\conf\extra) so your localhost can use the self-signed SSL certificate that we generated.
Edit the httpd-ssl.conf as below.
DocumentRoot "e:/wamp64/www"
ServerName localhost:443
ServerAdmin admin@example.com
ErrorLog "${SRVROOT}/logs/error.log"
TransferLog "${SRVROOT}/logs/access.log"
SSLSessionCache "shmcb:${SRVROOT}/logs/ssl_scache(512000)"
SSLCertificateFile "${SRVROOT}/conf/key/certificate.crt"
SSLCertificateKeyFile "${SRVROOT}/conf/key/private.key"
CustomLog "${SRVROOT}/logs/ssl_request.log" \
"%t %h %{SSL_PROTOCOL}x %{SSL_CIPHER}x \"%r\" %b"
DocumentRoot is where your document root of WampServer.
Restart WampServer
You must restart WampServer to take the effect.
After restart, the WampServer icon should be green. If not, you can right-click at the WampServer icon then select Tools and select “Check httpd.conf syntax“. In order to correct the syntax. After fixing the issue, restart WampServer again.
So now your localhost should be able to use HTTPS.
Additional
How to create a new project with SSL setting
In my case, I already had many projects before implementing the self-signed certificate. Those projects are set as visual hosts. They still can use “http://” as normal but they can not use “https://” yet. In order to use “https://” for them, I need to add the virtual host for them in httpd-ssl.conf.
Here is my example. Let’s say I want to add a new project on WampServer. Below are my steps.
- Add new Virtual Host for my new project. In my case, the new project is yellowdev.local. (If you use Windows, make sure you disable the antivirus to protect Windows hosts files from changes before adding new Virtual Host via WampServer. You will enable it back when your new Virtual Host is created.)
- Once you create a new Virtual Host, restart WampServer to take the effect.
- Now you should visit “http://yellowdev.local“.
- Next, open httpd-ssl.conf (E:\wamp64\bin\apache\apache2.4.46\conf\extra). And add the code below under </VirtualHost> which is located at the end of file. Basically, we add the new visual host for HTTPS.
<VirtualHost *:443>
ServerName yellowdev.local
ServerAlias www.yellowdev.local
DocumentRoot "e:/wamp64/www/yellowdev/web"
SSLEngine on
SSLCertificateFile "${SRVROOT}/conf/key/certificate.crt"
SSLCertificateKeyFile "${SRVROOT}/conf/key/private.key"
<Directory "e:/wamp64/www/yellowdev/web/">
SSLOptions +StdEnvVars
Options +Indexes +Includes +FollowSymLinks +MultiViews
Require all granted
AllowOverride All
</Directory>
BrowserMatch "MSIE [2-5]" \
nokeepalive ssl-unclean-shutdown \
downgrade-1.0 force-response-1.0
CustomLog "${SRVROOT}/logs/ssl_request.log" \
"%t %h %{SSL_PROTOCOL}x %{SSL_CIPHER}x \"%r\" %b"
</VirtualHost>
- Finally, restart WampServer to take the effect.
- Now you should visit “https://yellowdev.local“.
If you can not communicate with external API, follow this steps.
In my case, my project uses CraftCMS and the images are stored in AWS S3. The issue I have is no images are shown on the front end. I see the error from the browser console that says “Image transform cannot be created with HTTP 500 – Internal Server Error“. Meaning the project can not communicate with the external source which is AWS S3 in my case.
Then I check the image on the backend, I view the image and the image shows up but the URL comes from the CloudFront CDN. Then I download the image and I got the error that says “cURL error 60: SSL certificate problem: unable to get local issuer certificate“. Yes, I know the self-signed certificate is not complete.
To fix the incomplete SSL certificate, follow the steps below.
- Download the cacert.pem from this link. It is a zip file. Extract the file you will have a cacert.pem.
- Navigate to your WampServer folder (Xampp or MAMP that you use) and go to your “php\extras” folder. Then create a new “ssl” folder. Mine looks like this “E:\wamp64\bin\php\php7.4.24\extras\ssl“.
- Move the cacert.pem you just download into the “ssl” folder.
- Next, open your php.ini and search for “curl.cainfo“. Note that, you will need to update the php.ini from two places. One is at Wampserver>PHP {version you currently activates}>php.ini. This php.ini will remove the “cURL error 60” when you view the frameworks(eg: CraftCMS) on the browser. Another place is “E:\wamp64\bin\php\php{version you currently activates}\php.ini“. This php.ini will remove the “cURL error 60” when you update the frameworks(eg: CraftCMS) via the terminal.
- You will see “;curl.cainfo“. You want to uncomment it and add the absolute path for the cacert.pem. Below is my example in php.ini.
curl.cainfo = "E:\wamp64\bin\php\php7.4.24\extras\ssl\cacert.pem"
- Finally, restart the WampServer to take the effect on your localhost. It should fix the cURL error 60 issue like mine.
- If you have multiple PHP versions on your environment, you should check the correct php version of the current project which requests to use AWS S3. It has a chance that you change the PHP version for other projects and forget to swtich back to the correct PHP version of the project that requests to use AWS S3. It just happens to me today. lol.
Wrap up
How to configure the self-signed certificate on the localhost is changed from time to time. I will try to come back and update it. Hope this post is helpful. Here are the reference links I use for this post. Tutorial 1, Tutorial 2, and Tutorial 3.